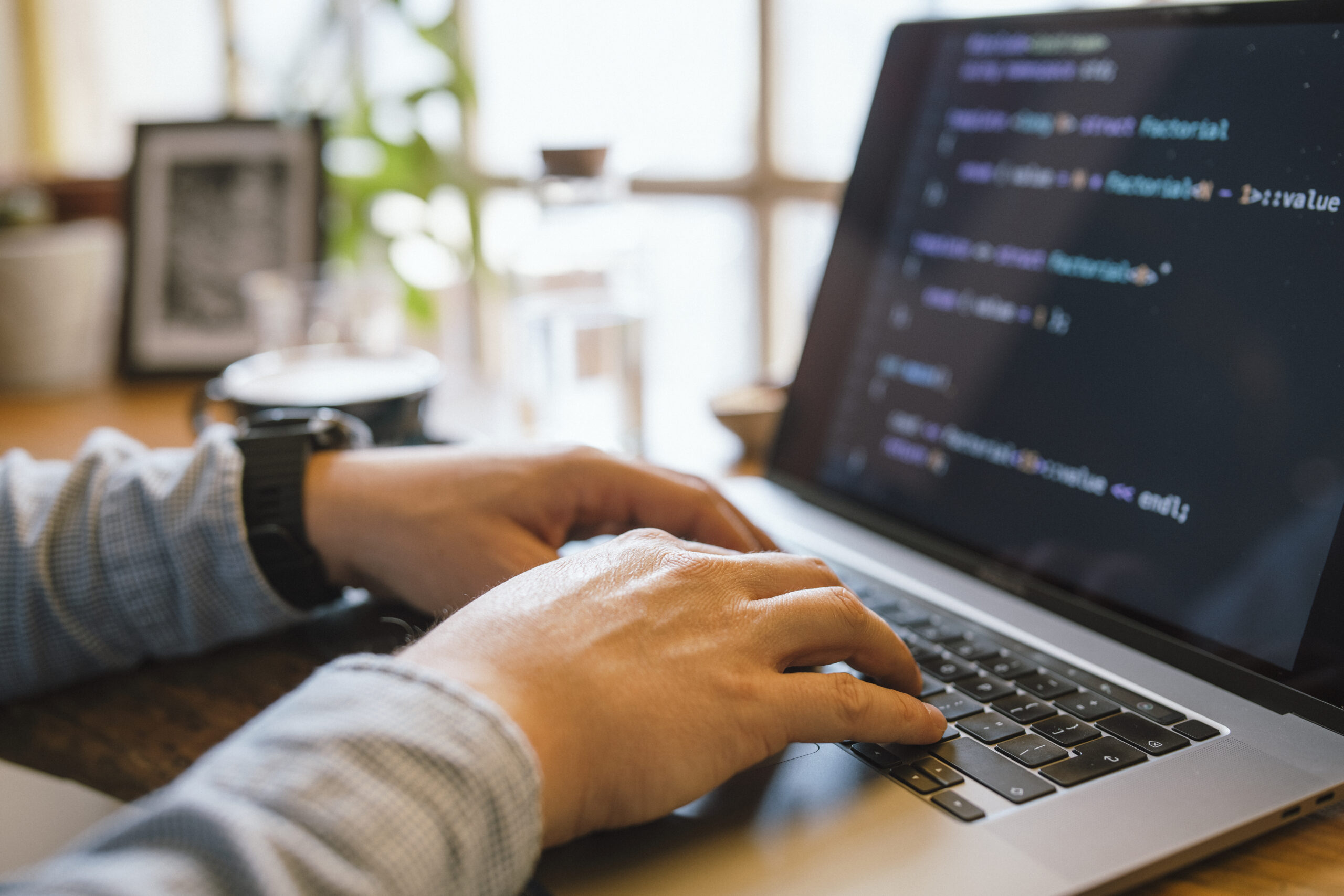
Debugging is Just about the most crucial — still often ignored — expertise in the developer’s toolkit. It isn't really nearly repairing broken code; it’s about comprehension how and why matters go Completely wrong, and learning to Consider methodically to resolve troubles successfully. Irrespective of whether you are a rookie or maybe a seasoned developer, sharpening your debugging abilities can conserve hours of frustration and significantly improve your productivity. Listed below are numerous techniques to assist developers amount up their debugging activity by me, Gustavo Woltmann.
Master Your Applications
On the list of fastest approaches developers can elevate their debugging skills is by mastering the resources they use each day. While crafting code is just one Section of advancement, figuring out the way to interact with it correctly through execution is equally important. Fashionable development environments appear Geared up with highly effective debugging abilities — but several developers only scratch the surface area of what these resources can perform.
Acquire, one example is, an Integrated Development Atmosphere (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These resources assist you to set breakpoints, inspect the worth of variables at runtime, action via code line by line, and even modify code over the fly. When utilised appropriately, they let you notice precisely how your code behaves for the duration of execution, which can be invaluable for tracking down elusive bugs.
Browser developer resources, which include Chrome DevTools, are indispensable for entrance-conclusion developers. They enable you to inspect the DOM, keep track of community requests, view true-time functionality metrics, and debug JavaScript in the browser. Mastering the console, resources, and community tabs can flip discouraging UI problems into manageable tasks.
For backend or process-level developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB provide deep control around operating procedures and memory administration. Understanding these applications might have a steeper Mastering curve but pays off when debugging functionality issues, memory leaks, or segmentation faults.
Over and above your IDE or debugger, come to be comfy with version Command devices like Git to comprehend code record, come across the exact minute bugs were launched, and isolate problematic modifications.
Eventually, mastering your instruments indicates heading further than default settings and shortcuts — it’s about developing an intimate expertise in your growth natural environment to ensure that when troubles crop up, you’re not dropped in the dead of night. The greater you know your equipment, the greater time you may invest fixing the particular challenge rather then fumbling through the process.
Reproduce the issue
One of the more critical — and often ignored — actions in successful debugging is reproducing the challenge. Ahead of jumping into your code or earning guesses, builders will need to make a regular setting or state of affairs the place the bug reliably appears. With out reproducibility, fixing a bug results in being a video game of probability, normally resulting in wasted time and fragile code modifications.
The first step in reproducing a dilemma is collecting as much context as you can. Inquire thoughts like: What steps resulted in The difficulty? Which natural environment was it in — growth, staging, or generation? Are there any logs, screenshots, or error messages? The greater depth you've, the easier it will become to isolate the exact situations below which the bug takes place.
As soon as you’ve gathered ample info, try to recreate the problem in your neighborhood atmosphere. This may indicate inputting the identical data, simulating very similar consumer interactions, or mimicking system states. If The problem seems intermittently, contemplate producing automated assessments that replicate the edge conditions or state transitions associated. These tests not merely help expose the challenge but additionally stop regressions Sooner or later.
Sometimes, the issue can be environment-certain — it'd come about only on sure operating programs, browsers, or less than specific configurations. Employing tools like virtual equipment, containerization (e.g., Docker), or cross-browser testing platforms may be instrumental in replicating these kinds of bugs.
Reproducing the condition isn’t just a stage — it’s a frame of mind. It demands persistence, observation, and also a methodical solution. But when you can constantly recreate the bug, you happen to be by now midway to repairing it. Using a reproducible circumstance, You should utilize your debugging applications extra successfully, examination opportunity fixes safely, and communicate much more Obviously using your staff or people. It turns an summary complaint right into a concrete obstacle — Which’s where by builders prosper.
Browse and Have an understanding of the Error Messages
Error messages tend to be the most respected clues a developer has when some thing goes Improper. As opposed to viewing them as discouraging interruptions, developers need to find out to deal with error messages as immediate communications within the procedure. They frequently tell you exactly what transpired, where by it took place, and often even why it happened — if you know the way to interpret them.
Start by examining the information diligently As well as in entire. A lot of developers, specially when under time tension, glance at the initial line and immediately start out generating assumptions. But deeper during the mistake stack or logs may possibly lie the accurate root induce. Don’t just copy and paste mistake messages into search engines like google and yahoo — read through and comprehend them very first.
Break the mistake down into parts. Could it be a syntax error, a runtime exception, or possibly a logic error? Does it level to a selected file and line variety? What module or function activated it? These questions can information your investigation and point you toward the accountable code.
It’s also practical to comprehend the terminology of your programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java frequently comply with predictable styles, and Finding out to recognize these can greatly quicken your debugging course of action.
Some errors are obscure or generic, As well as in those circumstances, it’s crucial to examine the context where the mistake occurred. Check out similar log entries, input values, and recent changes inside the codebase.
Don’t forget compiler or linter warnings possibly. These often precede greater troubles and supply hints about opportunity bugs.
Ultimately, mistake messages are usually not your enemies—they’re your guides. Studying to interpret them appropriately turns chaos into clarity, serving to you pinpoint challenges faster, decrease debugging time, and become a additional efficient and confident developer.
Use Logging Properly
Logging is The most highly effective applications in a developer’s debugging toolkit. When utilized successfully, it offers authentic-time insights into how an software behaves, encouraging you have an understanding of what’s going on underneath the hood without having to pause execution or move in the code line by line.
An excellent logging method begins with realizing what to log and at what degree. Typical logging ranges consist of DEBUG, INFO, WARN, ERROR, and FATAL. Use DEBUG for detailed diagnostic information and facts all through enhancement, Details for normal functions (like productive start out-ups), WARN for prospective problems that don’t break the applying, Mistake for real issues, and Lethal in the event the process can’t keep on.
Stay away from flooding your logs with excessive or irrelevant details. An excessive amount logging can obscure critical messages and slow down your procedure. Deal with critical activities, state variations, input/output values, and critical final decision factors in your code.
Structure your log messages Plainly and regularly. Involve context, for example timestamps, request IDs, and performance names, so it’s easier to trace difficulties in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
All through debugging, logs Allow you to keep track of how variables evolve, what situations are achieved, and what branches of logic are executed—all with no halting This system. They’re Specifically useful in output environments in which stepping as a result of code isn’t achievable.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
Eventually, smart logging is about equilibrium and clarity. Having a very well-thought-out logging strategy, you could reduce the time it will require to identify problems, attain deeper visibility into your programs, and Increase the overall maintainability and dependability of your respective code.
Think Like a Detective
Debugging is not only a complex activity—it is a method of investigation. To effectively recognize and correct bugs, builders must method the method similar to a detective solving a mystery. This attitude will help stop working elaborate issues into manageable components and stick to clues logically to uncover the foundation cause.
Begin by gathering evidence. Look at the signs of the challenge: mistake messages, incorrect output, or effectiveness challenges. Much like a detective surveys a crime scene, gather as much related details as you'll be able to with no jumping to conclusions. Use logs, examination circumstances, and consumer stories to piece jointly a transparent image of what’s taking place.
Up coming, type hypotheses. Inquire your self: What might be causing this conduct? Have any modifications lately been made to the codebase? Has this problem happened right before less than identical situation? The objective would be to slender down alternatives and establish likely culprits.
Then, check your theories systematically. Attempt to recreate the problem inside of a managed setting. When you suspect a certain operate or component, isolate it and validate if The problem persists. Like a detective conducting interviews, ask your code issues and Allow the results lead you nearer to the truth.
Pay back near interest to compact information. Bugs frequently disguise inside the least envisioned spots—like a missing semicolon, an off-by-one error, or a race affliction. Be thorough and client, resisting the urge to patch the issue devoid of entirely comprehending it. Momentary fixes might cover the real dilemma, just for it to resurface later.
And lastly, maintain notes on That which you experimented with and learned. Just as detectives log their investigations, documenting your debugging system can conserve time for long run problems and support others realize your reasoning.
By wondering like a detective, developers can sharpen their analytical competencies, method troubles methodically, and come to be more effective at uncovering hidden difficulties in elaborate methods.
Publish Assessments
Crafting assessments is among the simplest ways to boost your debugging capabilities and Over-all development efficiency. Tests not merely support capture bugs early but additionally serve as a safety net that provides you self confidence when building variations towards your codebase. A well-tested application is easier to debug since it lets you pinpoint just wherever and when a challenge takes place.
Begin with device exams, which target specific features or modules. These tiny, isolated assessments can promptly expose no matter whether a certain piece of logic is Functioning as anticipated. Whenever a test fails, you immediately know where to look, considerably decreasing the time used debugging. Device exams are Particularly helpful for catching regression bugs—issues that reappear just after Earlier currently being set.
Following, integrate integration checks and conclusion-to-stop tests into your workflow. These assistance be sure that a variety of elements of your software operate with each other smoothly. They’re particularly handy for catching bugs that arise in complicated units with a number of components or products and services interacting. If anything breaks, your tests can inform you which Portion of the pipeline unsuccessful and beneath what disorders.
Producing tests also forces you to definitely Believe critically regarding your code. To test a attribute correctly, you would like to comprehend its inputs, envisioned outputs, and edge circumstances. This level of understanding In a natural way leads to higher code composition and fewer bugs.
When debugging a concern, crafting a failing examination that reproduces the bug is usually a powerful initial step. Once the examination fails continuously, you may focus on repairing the bug and enjoy your test move when The problem is fixed. This strategy makes here certain that the same bug doesn’t return Later on.
Briefly, crafting assessments turns debugging from the frustrating guessing recreation into a structured and predictable course of action—helping you catch a lot more bugs, speedier plus more reliably.
Consider Breaks
When debugging a tricky concern, it’s uncomplicated to become immersed in the issue—watching your display screen for hrs, seeking solution following Remedy. But The most underrated debugging instruments is actually stepping absent. Getting breaks can help you reset your intellect, decrease disappointment, and sometimes see The problem from a new viewpoint.
When you're as well close to the code for as well lengthy, cognitive fatigue sets in. You may begin overlooking apparent problems or misreading code that you just wrote just hrs previously. Within this state, your Mind results in being fewer economical at challenge-fixing. A short walk, a espresso crack, or simply switching to another undertaking for ten–15 minutes can refresh your concentrate. Many builders report acquiring the basis of an issue after they've taken the perfect time to disconnect, allowing their subconscious perform within the background.
Breaks also assist prevent burnout, In particular through more time debugging periods. Sitting down before a display, mentally trapped, is not simply unproductive but additionally draining. Stepping absent means that you can return with renewed Vitality along with a clearer attitude. You might quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
For those who’re caught, a good general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–ten moment split. Use that point to move all-around, stretch, or do a little something unrelated to code. It might experience counterintuitive, Specifically less than restricted deadlines, but it really truly causes more quickly and more practical debugging In the long term.
In short, getting breaks is not a sign of weak point—it’s a sensible method. It offers your Mind Area to breathe, enhances your perspective, and aids you steer clear of the tunnel eyesight that often blocks your progress. Debugging is a mental puzzle, and relaxation is an element of solving it.
Find out From Each individual Bug
Each bug you come across is a lot more than just a temporary setback—It truly is a possibility to grow like a developer. Irrespective of whether it’s a syntax error, a logic flaw, or possibly a deep architectural difficulty, each one can teach you one thing precious for those who make an effort to mirror and assess what went Completely wrong.
Start by asking your self a number of critical issues as soon as the bug is fixed: What triggered it? Why did it go unnoticed? Could it are already caught previously with greater techniques like device screening, code opinions, or logging? The solutions generally expose blind places with your workflow or knowledge and make it easier to Make much better coding behaviors going ahead.
Documenting bugs will also be a wonderful pattern. Continue to keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, how you solved them, and Everything you discovered. As time passes, you’ll start to see styles—recurring difficulties or prevalent problems—which you can proactively stay away from.
In group environments, sharing Whatever you've realized from a bug with all your friends could be Particularly powerful. Irrespective of whether it’s by way of a Slack message, a brief publish-up, or a quick knowledge-sharing session, serving to Other folks avoid the exact situation boosts group effectiveness and cultivates a much better Mastering tradition.
Extra importantly, viewing bugs as lessons shifts your mindset from annoyance to curiosity. As opposed to dreading bugs, you’ll start appreciating them as necessary elements of your enhancement journey. All things considered, a few of the finest developers are certainly not the ones who produce ideal code, but individuals that repeatedly discover from their faults.
In the end, Just about every bug you repair provides a brand new layer on your skill set. So upcoming time you squash a bug, take a second to replicate—you’ll come away a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging techniques will take time, observe, and patience — even so the payoff is large. It helps make you a far more economical, confident, and capable developer. The subsequent time you might be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be better at Whatever you do.